Forcing ChatGPT to output whatever you want.
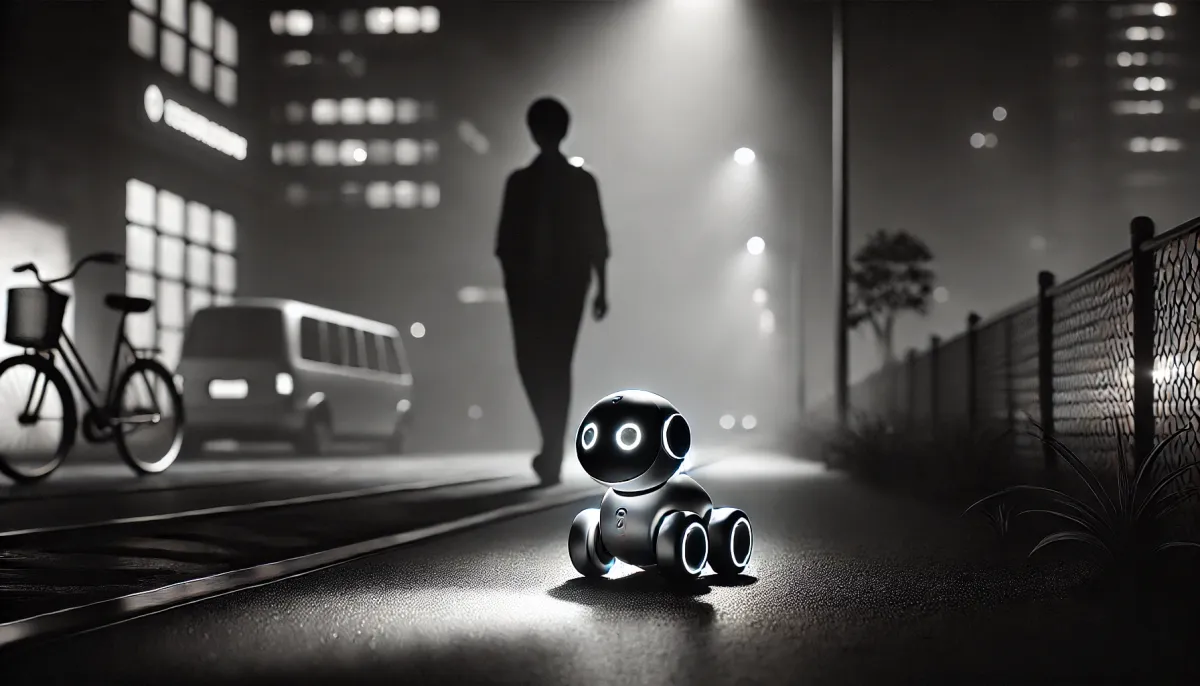
Using OpenAI, Zod, and Zod-to-JSON-Schema to Structure ChatGPT's Output
In this guide, we’ll go over how to use OpenAI with Zod and zod-to-json-schema
to enforce structured outputs from ChatGPT. With this setup, you’ll gain more control over the data format by defining an exact JSON schema.
Step 1: Import the Necessary Libraries
First, import OpenAI, Zod for schema validation, and zod-to-json-schema
to convert our Zod schema into JSON.
import OpenAI from 'openai';
import { z } from 'zod';
import { zodToJsonSchema } from 'zod-to-json-schema';
Step 2: Define the Output Schema with Zod
Let’s create a Zod schema that specifies our desired output structure. In this example, we’ll have joke
as a string and reasons
as an array of strings explaining why the joke is funny.
const jokeSchema = z.object({
joke: z.string().describe("A joke"),
reasons: z.array(z.string()).describe("Reasons why the joke is funny"),
});
Step 3: Convert the Zod Schema to JSON Schema
With zod-to-json-schema
, we’ll convert our Zod schema into JSON format, which can be used as part of the OpenAI API call.
const jokeSchemaJSON = zodToJsonSchema(jokeSchema);
const formattedSchema = JSON.stringify(jokeSchemaJSON); // Convert to JSON string
Step 4: Set Up the OpenAI Chat Completion Using the Updated API Call
Using the latest OpenAI API syntax, we’ll pass in the model, messages, and our JSON schema as part of the function call. This ensures OpenAI’s response aligns with our defined structure.
async function main() {
const completion = await openai.chat.completions.create({
model: "gpt-4",
messages: [
{ role: "system", content: "You are a joke teller that outputs jokes in JSON format." },
{ role: "user", content: "Tell me a joke about dogs and cars." }
],
function_call: {
name: "generateJSON",
parameters: formattedSchema,
},
});
console.log(completion.choices[0].message.content);
}
Step 5: Run and Verify the Output
Once you run this code, you should receive a response from OpenAI that matches your schema, outputting the joke and reasons in JSON format.
main().catch(console.error);
Conclusion
Combining OpenAI with Zod and zod-to-json-schema
gives you precise control over response formatting, ensuring you receive structured JSON outputs. This setup is perfect for applications requiring reliable, predictable data, and it simplifies handling responses programmatically. Give it a try and enjoy structured outputs tailored to your needs!